How to build a Real-Time Messaging App using Diffusion
September 15, 2020 | Matias Prados
Real-Time Messaging
Introduction to Diffusion Real-Time Messaging through a simple application using Diffusion Cloud.
A set of simple projects, illustrating production and consumption of messages to and from a Diffusion Cloud instance.
These JavaScript code examples enable users to interact in real-time across various rooms or topics. You can also use other programming languages from our SDKs, including iOS, Android, C, .NET, and more.
Lesson 1: Publish and Subscribe to Topics
Lesson 1 code example introduces the concept of Publish and Subscribe to ‘Topics’ (data structures). In Diffusion, data is stored and distributed through Topics.
Lesson 2: Time Series Topics
Lesson 2 code example introduces the concept of Time Series topic to hold a historical sequence of events. Time series topics are useful for collaborative applications such as chat rooms. Multiple users can concurrently update a time series topic.
Lesson 3: Security
Lesson 3 code example introduces the concept of Security by requiring sessions to authenticate and use role-based authorization to define the actions that a client can perform.
Pre-requisites
- Download our code examples or clone them to your local environment:
- A Diffusion service (Cloud or On-Premise), version 6.5.0 or greater. Create a service here.
- Follow our Quick Start Guide and get your service up in a minute!
Setup
Make sure to add Diffusion library to your code. For JavaScript, we have added the following line in our public/chat.html
:
Set lines 44-46 of public/js/app.js
to the hostname of your Diffusion Cloud service, which you can find in your service dashboard. You can also leave the default values and connect to our sandbox service:
- host: host (“diffusionchatapp.eu.diffusion.cloud” by default)
- user: ‘user’
- password: ‘password’
Execution
Really easy, just open the index.html file locally and off you go!
The code in action
(Click to watch the video)
Lesson 1: Publish and Subscribe
In Diffusion, data is stored and distributed through ‘Topics’ (data structures). Session can subscribe to a topic to receive notifications when the topic value changes and can also update the value. When a topic is updated, all its subscribers are notified of the new value. Diffusion takes care of efficiently broadcasting value changes, even if there are hundreds of thousands of subscribers.
Step 1: Connect to Diffusion
diffusion.connect > create your host
diffusion.connect({
host : host, // Use your Diffusion service or connect to our sandbox "diffusionchatapp.eu.diffusion.cloud"
principal : "user",
credentials : "password"})
Step 2: Create a Topic
session.topics.add
session.topics.add(_roomTopic, diffusion.topics.TopicType.JSON);
Step 3: Create a Topic Listener
session.addStream
session.addStream(_roomTopic, diffusion.datatypes.json());
Step 4: Subscribe to a Topic
session.select
session.select(_roomTopic);
Step 5: Update a Topic
session.topicUpdate.set
session.topicUpdate.set(_roomTopic, diffusion.datatypes.json(), { text: msg, name: name, timestamp: new Date().toLocaleTimeString() });
Lesson 2: Time Series
Introduce the concept of a Time Series topic to hold a sequence of events. Time series topics are useful for collaborative applications such as chat rooms. Multiple users can concurrently update a time series topic.
Step 1: Topic Types and Specifications
diffusion.topics.TopicSpecification : TopicType.TIME_SERIES
diffusion.topics.TopicSpecification(diffusion.topics.TopicType.TIME_SERIES,
{
TIME_SERIES_EVENT_VALUE_TYPE : "json",
TIME_SERIES_RETAINED_RANGE: "limit 100",
TIME_SERIES_SUBSCRIPTION_RANGE: "limit 100"
});
Step 2: Append/Update Time Series
session.timeseries.append
session.timeseries.append(_roomTopic,
{
text: msg
name: name,
timestamp: new Date().toLocaleTimeString()
},
diffusion.datatypes.json());
Lesson 3: Security
Introduce the concept of Security by requiring sessions to authenticate and use role-based authorization to define the actions that a client can perform.
Step 1: Using Auth script builder
session.security.authenticationScriptBuilder()
session.security.authenticationScriptBuilder();
roles
Step 2: Create a new user with a user name, password andaddPrincipal() – build()
authenticationScriptBuilder
.addPrincipal(name, password, ['CLIENT','TOPIC_CONTROL'])
.build();
Step 3: Update the Auth store
session.security.updateAuthenticationStore()
session.security.updateAuthenticationStore(addUserScript);
Challenge for you
Now that you have a fully functional messaging app, try building a “moderator” app, to monitor the conversation for explicit/inappropriate messages. When specific text is identified in a conversation, the message value can be bleeped out, or access/permission to the room can be dynamically updated based on the user’s behavior.
Watch our Webinar
Tuned our webinar introducing the basic concept of your Diffusion dashboard and console.
Thank you!
Further reading
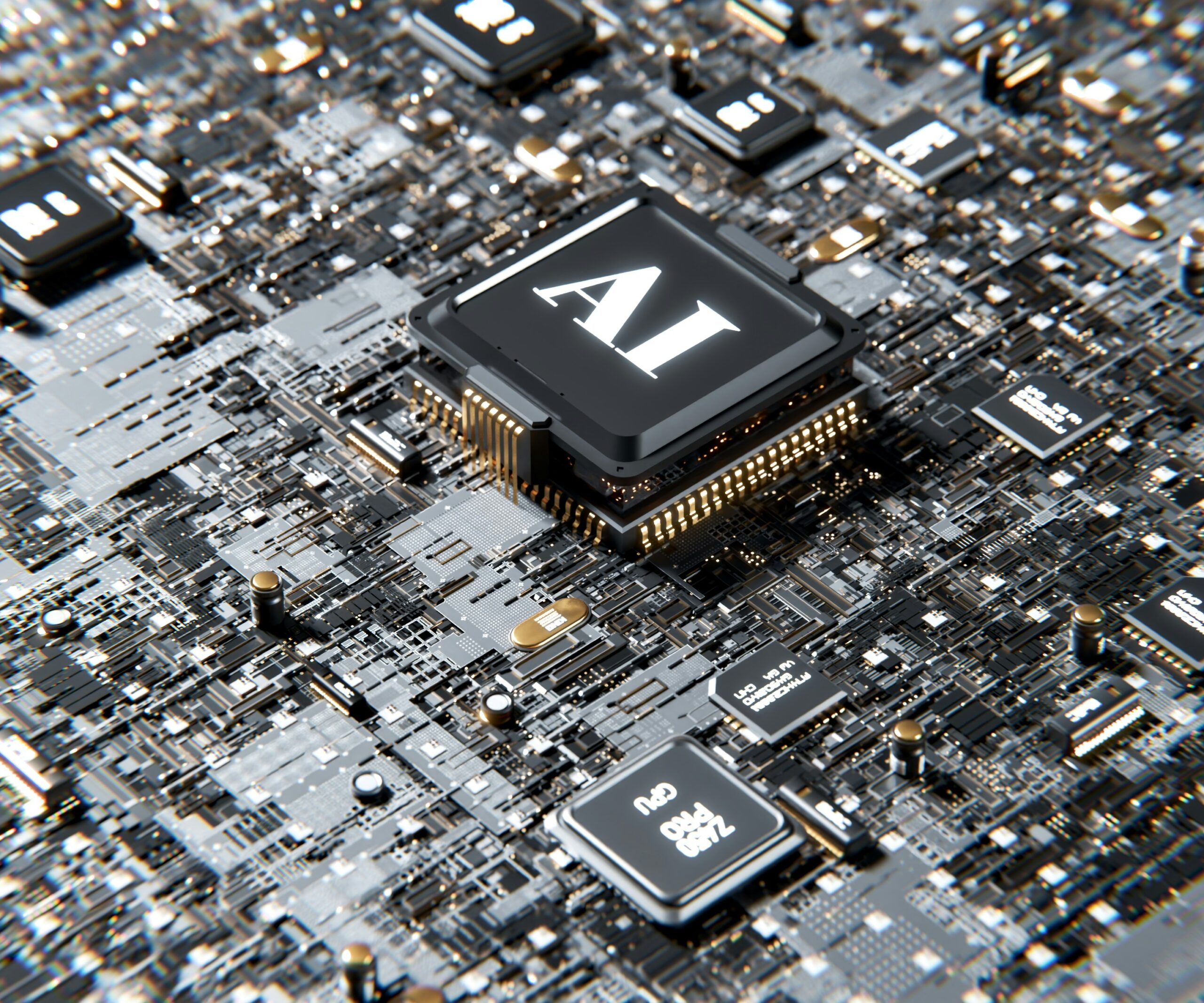
BLOG
Exploring Generative AI: Opportunity or Potential Headache?
March 25, 2024
Read More about Exploring Generative AI: Opportunity or Potential Headache?/span>
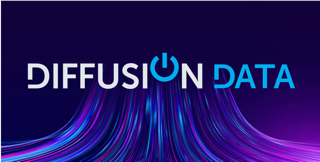
BLOG
100 million updates per second - Landmark Diffusion cluster performance
July 02, 2024
Read More about 100 million updates per second - Landmark Diffusion cluster performance/span>
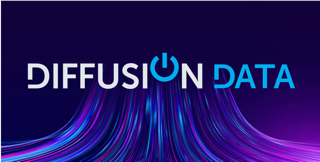
BLOG
Benchmarking and scaling subscribers
March 15, 2024